Table Of Content
- Introducing Factory Method
- Design Patterns
- Basic Implementation of Factory Method
- Factory Design Pattern
- Advantages of the Factory Method Design Pattern
- From Concept to Creation: Understanding Factory Design
- The Art of Decorating: Applying the Decorator Design Pattern in Real L...
- Factory Method Design Pattern Example in Java
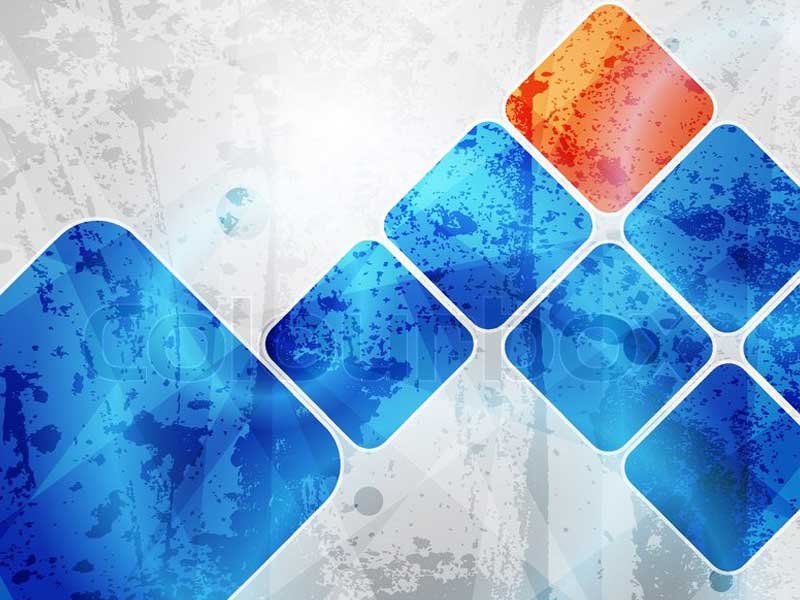
Finally, we call the draw() method on these objects, which produces the expected output. Client Code is nothing but the class from where we need to consume the product classes (MoneyBack, Titanium, and Platinum). And in our example, it will be the Main method of the Program class. We will ask the user to select the Credit Card Type in the client code. The application defines a config dictionary representing the application configuration.
Introducing Factory Method
Instead of knowing the exact object class and instantiating it through a constructor, the responsibility of creating an object is moved away from the client. So, we have created three Product classes that implement the CreditCard interface. Next, we must consume the Product classes inside the client code by creating and initializing the appropriate Product class object.
Design Patterns
Now let's explore the ways to implement the Factory design pattern together. From the class diagram, coders can easily see that the implementation for Factory is extremely straightforward. AccountFactory takes an AccountType as input and returns a specific BankAccount as output. The main idea is to define an interface or abstract class (a factory) for creating objects. Though, instead of instantiating the object, the instantiation is left to its subclasses.
Basic Implementation of Factory Method
The registration information is stored in the _creators dictionary. The .get_serializer() method retrieves the registered creator and creates the desired object. If the requested format has not been registered, then ValueError is raised. The Song class implements the Serializable interface by providing a .serialize(serializer) method.
Building a Multi-Tenant SaaS Solution Using Amazon EKS Amazon Web Services - AWS Blog
Building a Multi-Tenant SaaS Solution Using Amazon EKS Amazon Web Services.
Posted: Thu, 04 Mar 2021 08:00:00 GMT [source]
This component evaluates the value of format and returns the concrete implementation identified by its value. On the other hand, the Abstract Factory pattern gives us a way to make groups of related or dependent objects without having to specify their concrete classes. It encapsulates a group of factories, each of which creates a family of objects that are related to each other. In the above code you can see the creation of one interface called IPerson and two implementations called Villager and CityPerson. Based on the type passed into the PersonFactory object, we are returning the original concrete object as the interface IPerson.
Advantages of the Factory Method Design Pattern
The framework receives the shape type as a string parameter, it asks the factory to create a new shape sending the parameter received from menu. The factory creates a new circle and returns it to the framework, casted to an abstract shape. Then the framework uses the object as casted to the abstract class without being aware of the concrete object type. Room is the base class for a final product (MagicRoom or OrdinaryRoom).
From Concept to Creation: Understanding Factory Design
The example above exhibits all the problems you’ll find in complex logical code. Complex logical code uses if/elif/else structures to change the behavior of an application. Using if/elif/else conditional structures makes the code harder to read, harder to understand, and harder to maintain. It separates the process of creating an object from the code that depends on the interface of the object.
For example, your application might require in the future to convert the Song object to a binary format. This interface is implemented by the concrete classes JsonSerializer and XmlSerializer. Pentalog is a digital services platform dedicated to helping companies access world-class software engineering and product talent. With a global workforce spanning 16 locations, our staffing solutions and digital services power client success.
How to use fluent interfaces and method chaining in C# - InfoWorld
How to use fluent interfaces and method chaining in C#.
Posted: Mon, 07 Sep 2020 07:00:00 GMT [source]
Wikipedia has a good catalog of design patterns with links to pages for the most common and useful patterns. Also, Spotify and Pandora require an authorization process before the service instance can be created. The implementation of SerializerFactory is a huge improvement from the original example. It provides great flexibility to support new formats and avoids modifying existing code. By implementing Factory Method using an Object Factory and providing a registration interface, you are able to support new formats without changing any of the existing application code. This minimizes the risk of breaking existing features or introducing subtle bugs.
Specifically, the Factory Method and Abstract Factory are very common in Java software development. Pranaya Rout has published more than 3,000 articles in his 11-year career. Let us first see the example without using the Factory Design Pattern. Still, the current implementation is specifically targeted to the serialization problem above, and it is not reusable in other contexts.
This example is short and simplified, but it still has a lot of complexity. There are three logical or execution paths depending on the value of the format parameter. This may not seem like a big deal, and you’ve probably seen code with more complexity than this, but the above example is still pretty hard to maintain. Adding a new class to the program isn’t that simple if the rest of the code is already coupled to existing classes.
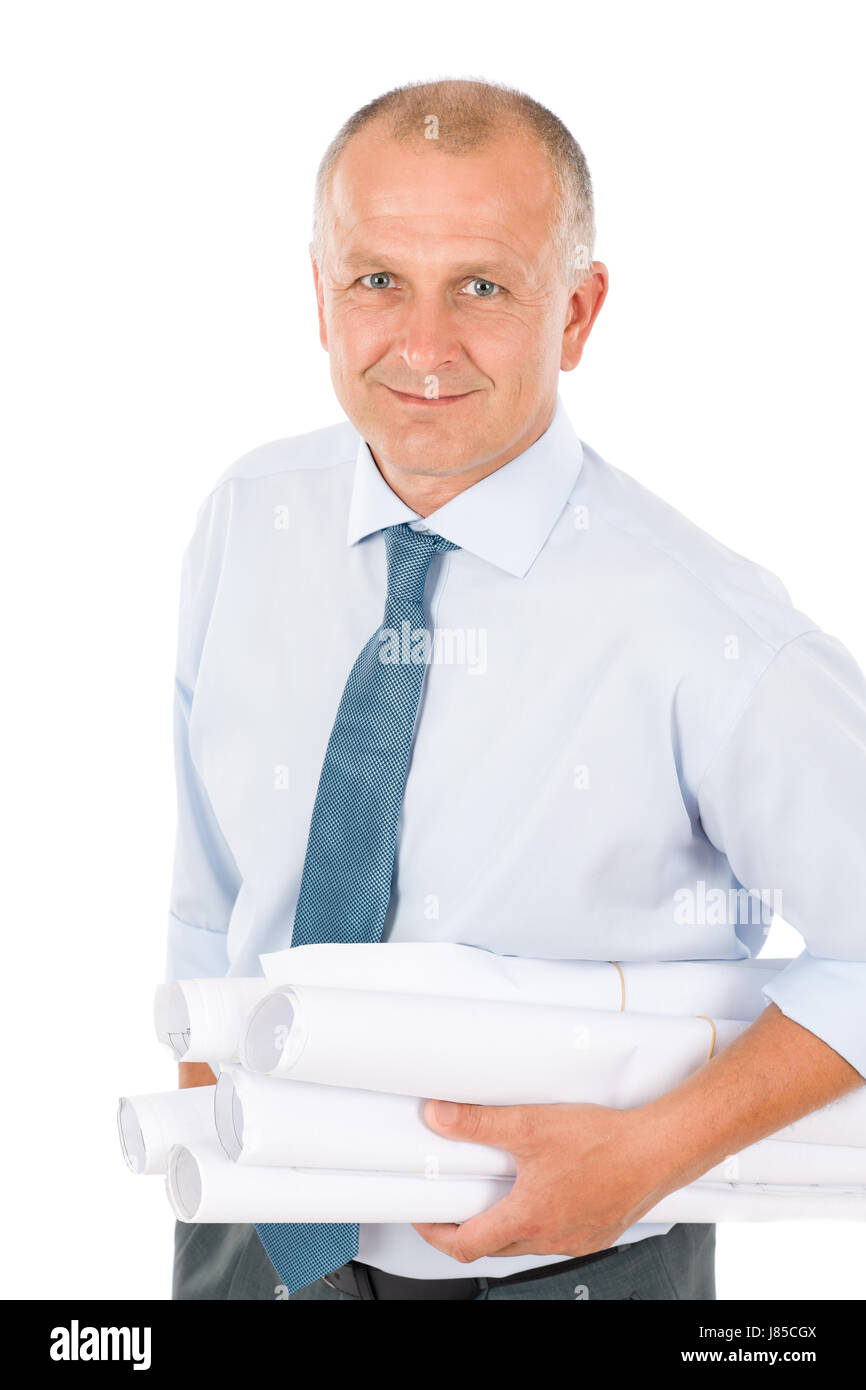
That method will create and return different objects based on the received input parameter“. In this article, I will discuss the Factory Design Pattern in C# with Examples. The Factory Design Pattern is one of the most frequently used design patterns in real-time applications.
No comments:
Post a Comment